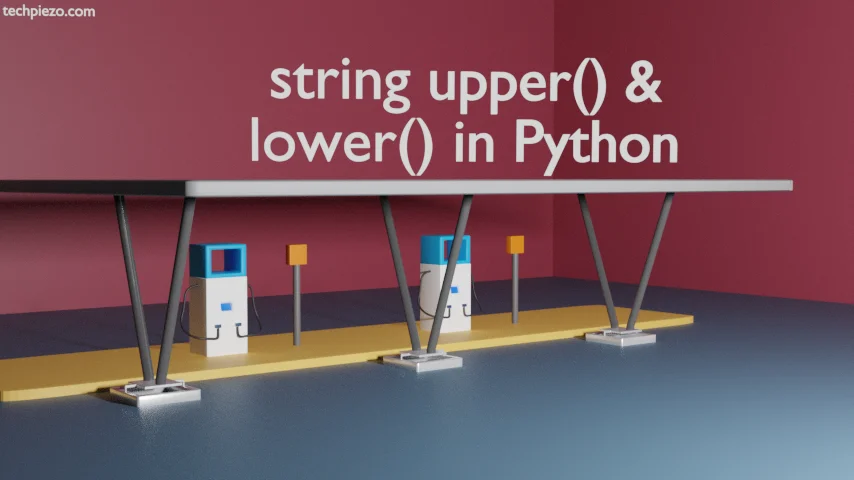
In this article, we would cover string upper() & lower() method in Python. string upper() method is used to return a string which contain all uppercase characters. where, string lower() would return a string which contains all the lowercase characters.
The syntax for string upper() method is –
str.upper()
And, the syntax for string lower() method is –
str.lower()
We understand the above with appropriate examples next.
string upper() in Python
We start with string upper() method. Let’s say we have a variable, x which contains a string –
x="Hello World!"
Now, to convert all the characters stored in variable x to uppercase –
outcome=x.upper()
We store the result in variable outcome. To print the result –
print(outcome)
It would return with –
HELLO WORLD!
string lower() in Python
We continue with the above example. Variable x stores string values –
x="Hello World!"
To convert all the characters stored in variable x to lowercase –
outcome=x.lower()
Again, we stored the result in variable outcome. Lastly, to print the result –
print(outcome)
It would return with the following –
hello world!
While using both upper() and lower() methods, you would have noticed that we have exclamation mark (!) there in the string. Still no warnings were received while using both the methods. Reason – both these methods ignore symbols and numbers.
In conclusion, we have covered string upper() & lower() method in Python.
Additional Info –
We can also check whether a string contains only upper-case or lower-case letters. This can be done through isupper() or islower() method. If all the characters in a string contains upper-case and lower-case characters then only, it would return with True. Otherwise, if it contains even one lower-case or upper-case characters then it would return with False.
Let’s understand with the following examples –
Example I. We have a variable x –
x="Hello World!"
To check for isupper() –
print(x.isupper())
It returns with –
False
Similarly, it would return with False again if we check for islower(). Because, not all characters are lower-case.
Example II. To check for isupper() –
x="HELLO WORLD!" print(x.isupper())
It returns with –
True
Similarly, for islower() –
x="hello world!" print(x.islower())
It returns with –
True