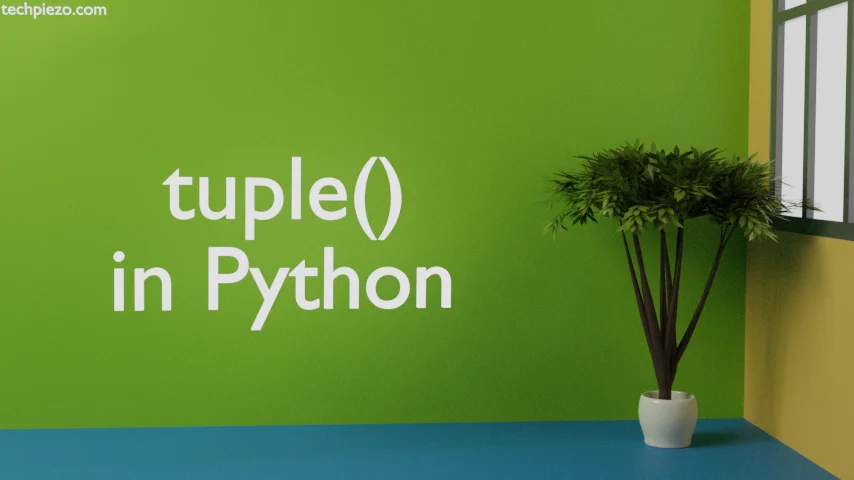
In this article, we would cover tuple() method in Python. This is different from the sequence data type – tuple, which we have already discussed. tuple() is basically a built-in function which is used to convert an input sequence into a tuple.
Although, it may seems trivial but, at times the tuple() method do comes in pretty handy.
tuple() method in Python
Use tuple() method in case we want create a tuple from an input sequence. This input sequence could be a list, dict, string etc. It is worth mentioning here that, the resultant tuple so obtained would contain the elements in the same order as that of input. It the input iterable is already a tuple then, it wouldn’t change anything.
Apart from that, if we don’t provide anything as input then, it would create an empty tuple.
Understand it more with the following examples.
Example I. Create a tuple from a list, x.
x = ['book', 'science', 9]
We want to convert it to a tuple and store the result in variable y.
y = tuple(x)
To get the output –
y
It would return with –
('book', 'science', 9)
Example II. Create a tuple from a dictionary, x.
x = {1: 'baseball', 2: 'rugby'}
Thereafter, store the tuple outcome in variable y.
y = tuple(x)
To view the output –
y
This would return with –
(1, 2)
Example III. Create a tuple from a string, x.
x = 'TechPiezo'
Next, store the resultant in variable y.
y = tuple(x)
For output –
y
It would return with –
('T', 'e', 'c', 'h', 'P', 'i', 'e', 'z', 'o')
Example IV. Lastly, what if we don’t provide anything as an input –
x = tuple()
To see the output –
x
It would return with –
()
In conclusion, we have covered tuple() method in Python here.