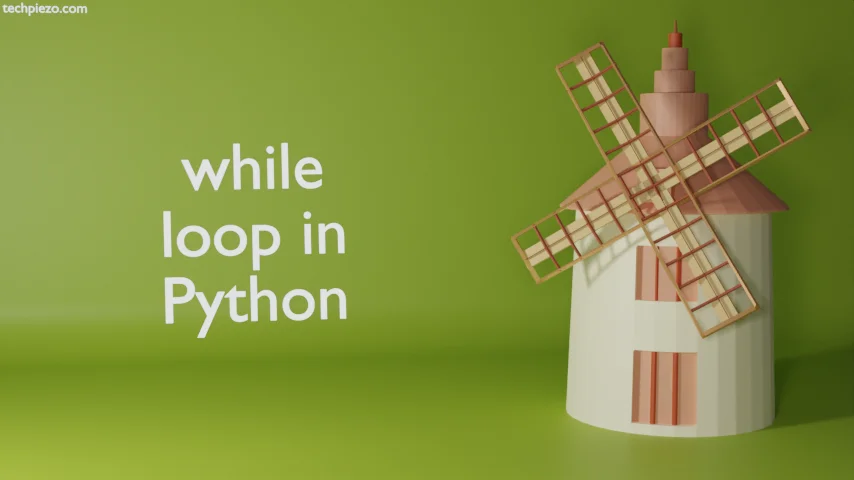
In this article, we would cover while loop in Python. When we want to execute a specific instruction set repeatedly, then in that case we use loop statements. while loop is one of the most preferred loop statement.
If we don’t use a loop statement then, we have to write the instruction set for every specific condition. And, this definitely isn’t the preferred option.
For while loop, we need to provide the condition for which our instruction set needs to be executed. And, in the body there would be instruction set itself.
The while loop syntax –
while condition: body
The condition in while loop is checked first. If the condition satisfies only then, it would move towards instruction set placed in body of while loop. Thereafter, it would keep checking for the condition till it is True. If the condition is not met – it exits from the loop.
We discuss while loop more with appropriate examples in next section.
while loop in Python
Example I. Generate a sequence of integers through while loop –
x = int(input("Enter value to generate an integer sequence: ")) while x > 0: print(x, end="\n") x = x-1
Note: We have used one tab space in while loop’s body consisting of print() method etc.
Here, we asked the user to provide the integer input to generate a sequence. The input from the user is stored in variable x. So, till the time x is greater than 0 – while loop will execute the instruction set provided in the body.
The above code will help us get a list of integers in descending order as per the input. So, if the input from the user is 4. Then, the output would be –
4 3 2 1
Example II. To get the sum for n natural numbers –
x = int(input("Enter value to get sum of n natural numbers: ")) sum = 0 while x > 0: sum = sum + x x = x - 1 print("The sum of natural numbers as per input is: ", sum)
Here, user is asked to provide the integer input. Let’s say the user provides the input: 4, then sum would be 4+3+2+1 = 10. We initialize the variable sum as zero. And, run while loop till the value of x is greater than zero. The outcome is stored in variable sum, which adds value of x with itself till the condition satisfies. At every run, the value of x is decreased by one.
In conclusion, we have discussed while loop in Python.