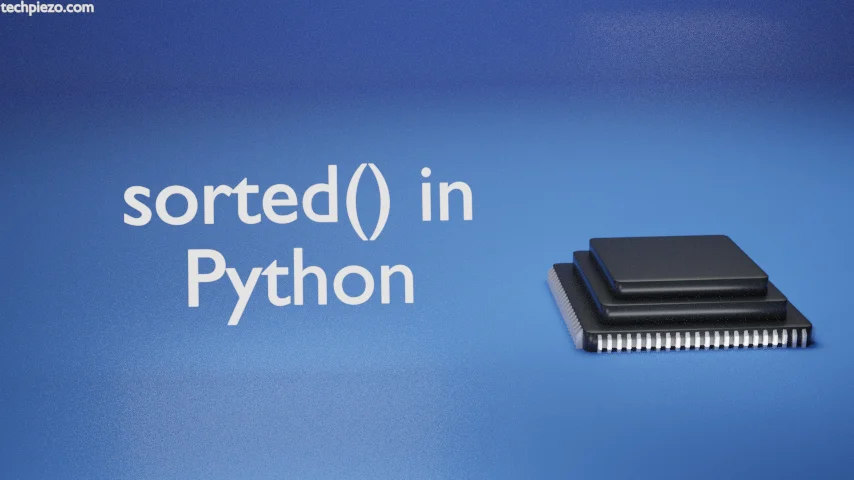
In previous article, we have discussed – sort and reverse sort in Python. That was specifically for lists. But, when it comes to sorting other iterables including lists. Then, we need to use built-in sorted() method. In this article, we would cover sorted() method in Python.
So, sorted() method basically sorts any iterable and returns with a list. By default, it returns a list in ascending order. We use sorted() when we want to retain the original iterable intact.
sorted() in Python
Now, let’s understand how it works with the help of an example. Let’s say we have a tuple, x –
x=("k","x","a")
Use sorted() method to sort –
y=sorted(x)
Lastly, to see the output –
y
This would return with –
['a', 'k', 'x']
As already discussed, the sorted() method by default sorts in an ascending order. To sort in descending order, we need to use reverse parameter and set it to True. By default, the reverse parameter is set to False.
Continuing with the above example –
x=("k","x","a")
To reverse sort with sorted() method –
y=sorted(x, reverse=True)
Thereafter, to print the output –
y
The output we see –
['x', 'k', 'a']
In conclusion, we have discussed how to use sorted() method in Python. Also, sorted() method can be put to work on any iterable. This iterable may include lists, tuples, dictionaries etc. Apart from that, sorted() method makes a new iterable. It doesn’t make changes to the original iterable.
We would like to add here that, we would explain it more through complex examples in coming articles.