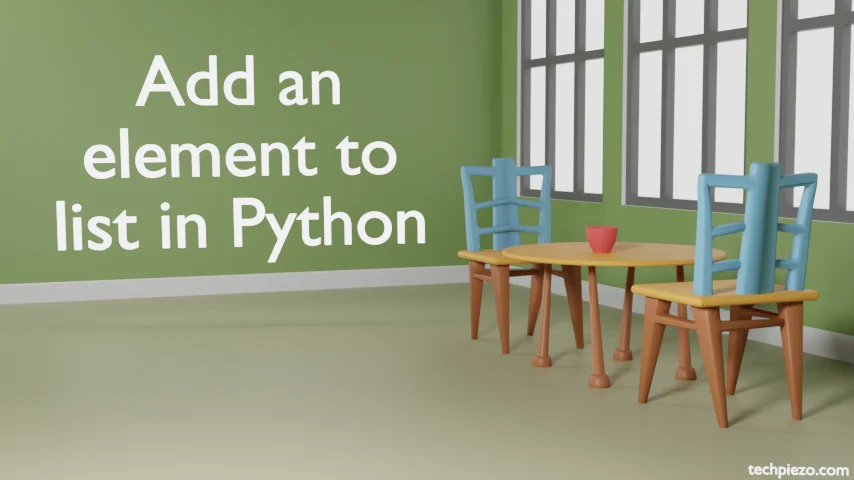
In this article, we would cover how to add an element to the list in Python. Adding an element to the list is pretty simple. We will discuss three methods here –
- append,
- insert and,
- extend.
Also, unlike tuples lists are mutable. Therefore, we can make necessary changes to the elements of list when required.
Add an element to the list in Python
Let’s say we have a list, x. Now, we will start with append first.
Method I. append() – as the term itself suggests, it helps us add an element to the end of the list.
x=[2,4,5,6]
To the add the element – 10 – at the end of the list –
x.append(10)
Use print() function to see the output –
print(x)
It would return with –
[2, 4, 5, 6, 10]
Method II. insert() – it is used to insert an element to the list at a specific index. Since, it requires an index number therefore, we need to provide the index number along-with the element which we want to insert. Let’s understand it with the following example –
x=[4,6,7,8]
Now, if we want to insert integer 10 at the index number 1.
x.insert(1, 10)
Use print() function, to understand what went behind the scenes –
print(x)
It would return with –
[4, 10, 6, 7, 8]
Here, insert() method basically helped us insert integer value – 10 at the index number 1. Notice how index numbers of other elements (i.e. 6,7,8) following it have shifted.
Method III. extend() – Let’s say we have got another list, y. And, now we want to combine it with x. So, we would use append() method. By using this method, we merely append the list y with list x. Following example would help us understand better –
x=[2,4,5,6] y=["a","b","c"]
To extend x with y –
x.extend(y)
Use print() function to get the output –
print(x)
This would return with –
[2, 4, 5, 6, 'a', 'b', 'c']
In conclusion, we have discussed how to add an element to the list in Python using append(), insert() and extend() methods.