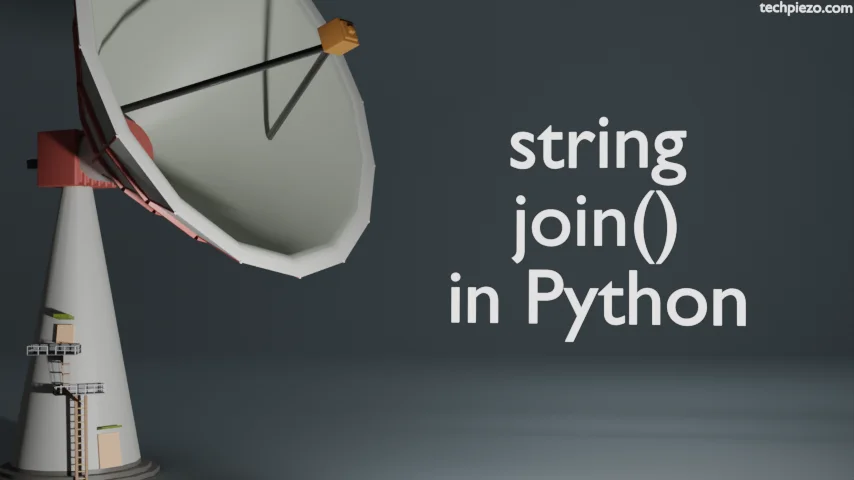
In this article, we would cover string join() method in Python. join() method mainly concatenates all the items of an iterable into a string. The syntax of the join() method is –
str.join(iterable)
It is worth mentioning here that, it will throw a TypeError if we try to join non-strings values in an iterable. In addition to, we always have to provide a separator.
We discuss join() method with appropriate examples next.
string join() in Python
Example I. We take the TypeError example first. Let’s say we have a list x, and it contains only integer values –
x=[2,5,6,7,8]
Now, to join it with hash (#) –
sep='#' outcome=sep.join(x)
We store the result in variable outcome. But, at this stage – it would throw an error –
TypeError: sequence item 0: expected str instance, int found
Reason – there are non-string values in the iterable. So, it returns with a TypeError, which says it expected str instance. Instead, all it found was integer values. In next example, we provide string values only.
Example II. We continue with the above example, just that this time around we provide string values –
x=['2','5','6','7','8'] sep='#' outcome=sep.join(x) print(outcome)
The output would be –
2#5#6#7#8
Example III. Since, we have used only lists up till now. So, for this example we chose to have a tuple – x
x=("Hello","How are you?","Bye") outcome='$'.join(x) print(outcome)
It would return with –
Hello$How are you?$Bye
You would notice in the code that, we haven’t used a variable for the separator. Instead, we chose to directly provide it. That is another way to use join() method. Besides, in the code we have used print() method to print the data stored in variable outcome.
In conclusion, we have discussed how to concatenate string values in an iterable into a string through join() method in Python. We consider it as inverse of split() method in Python. For more about spit() method in Python.