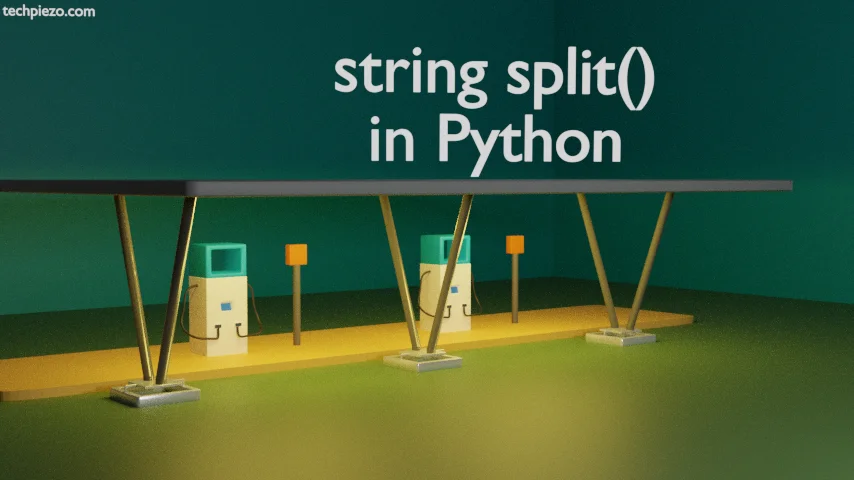
In this article, we would cover string split() method in Python. The split() method is used to split a string into a list. The following is the syntax of split() method –
str.split(separator, maxsplit)
where,
separator – empty space is the default separator. Otherwise, we use a separator like comma, hash etc. which splits the string. Besides, it is an optional parameter.
maxsplit – again an optional parameter. The default value is -1. The value shows how many splits it will do. If the default value, -1, is there then it will separate the string every time it encounters a separator till the end of string.
string split() in Python
Let’s understand the above with few examples.
Example I. If we don’t provide the separator and maxsplit values.
x="Hello World!" spt=x.split() print(spt)
Here, we stored a string “Hello World!” in variable x. Then, split the string with split() method and stored the result in variable spt. Lastly, the outcome was there to see through print() method.
It would return with –
['Hello', 'World!']
Since, we didn’t provide any separator. Therefore, it took default separator as empty space. The reason why Hello and World! were split up. In addition to, we also didn’t specify maxsplit as well. So, it chose to split every occurrence of the separator till the end of string.
Example II. This time, we choose to provide only the separator while sticking with default maxsplit value i.e. -1
x="Hello ABC, How have you been?, Bye" spt=x.split(',') print(spt)
Now, we won’t repeat the code explanation. We will just cover the mechanics of how things are working. We are taking comma (,) as a separator. It would return with –
['Hello ABC', ' How have you been?', ' Bye']
It separated the string based on every occurrence of comma(,) till the end of the string.
Example III. Here, we would consider both separator and maxsplit –
x="Hello ABC$ How have you been?$ Bye" spt=x.split('$', 1) print(spt)
We are taking $ as the separator and maxsplit value as 1. So, it should do just 1 split for the separator. When we run the above code, it would return with –
['Hello ABC', ' How have you been?$ Bye']
The above outcome is self-explanatory. The string split only for the first occurrence of the separator $ while rest of string stay as it is.
In conclusion, we have discussed how to string split() in Python.