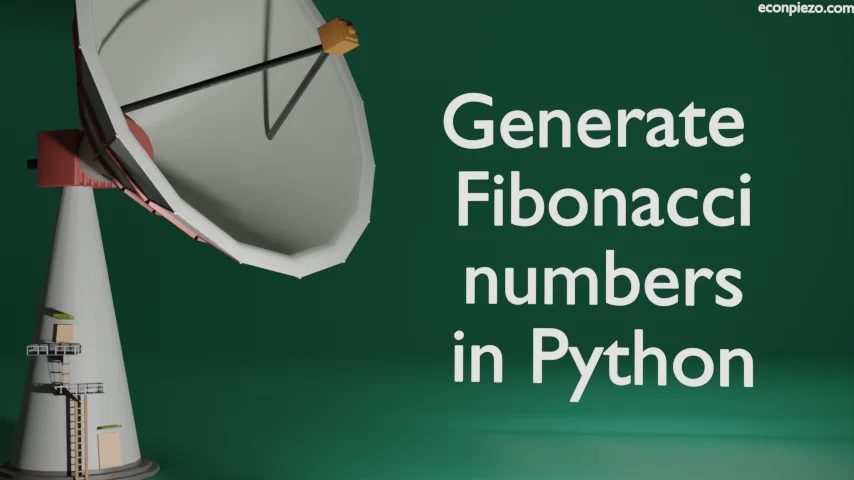
In this article, we cover how to generate Fibonacci numbers in Python.
Introduction
It is important to understand what Fibonacci numbers are. When each number in a sequence is the sum of two preceding numbers then it forms a Fibonacci sequence. And, the numbers that are part of such a sequence so formed as basically known as Fibonacci numbers.
Fibonacci Sequence:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34
Here, we can clearly see from the left that: 0 and 1 form the third number 1. And, 1 and 1 results in 2. Similarly, 2 and 3 result in 5 (the sixth number in sequence). The new number so formed is the sum of the preceding two numbers.
Python program that helps generate Fibonacci numbers
num = int(input("Enter how many fibonacci numbers you wish to generate: ")) fib_num = [] if num == 1: fib_num = [0] elif num == 2: fib_num = [0,1] else: fib_num = [0,1] for i in range(1,num-1): x = fib_num[i]+fib_num[i-1] fib_num.append(x) print(fib_num)
We used the input() function to ask the user to enter the Fibonacci numbers that they wish to generate and store the result in variable num.
Thereafter, we created an empty list: fib_num to store the Fibonacci numbers.
Then, there is a conditional statement: if-elif-else. If the number the user wishes to generate is 1 then, fib_num would have only zero. The same goes with if num == 2. There will be only two values: 0 and 1.
But, if there are more than two numbers then, we use a for loop. As we already know, the Fibonacci sequence will be a set of Fibonacci numbers that are the sum of the preceding two numbers. We already have two numbers with us i.e. 0 and 1. So, we need to cover the rest in the sequence.
fib_num[i] and fib_num[i-1] will cover the preceding two numbers. And, the resulting number is stored in variable x. The value in x is then appended to the fib_num through the append() function.
Lastly, we print the sequence of Fibonacci numbers through print(fib_num).
Testing the code
So, we run our program. It would prompt us:
Enter the number of fibonacci numbers you wish to generate: 10
And, we enter 10. This returns us a Fibonacci sequence list.
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Conclusion
In this article, we learned how to generate a list of Fibonacci numbers through a Python program. Fun fact: The Golden ratio is closely associated with the Fibonacci Sequence. If you would like to know more about the Golden ratio then:
1https://www.adobe.com/in/creativecloud/design/discover/golden-ratio.html