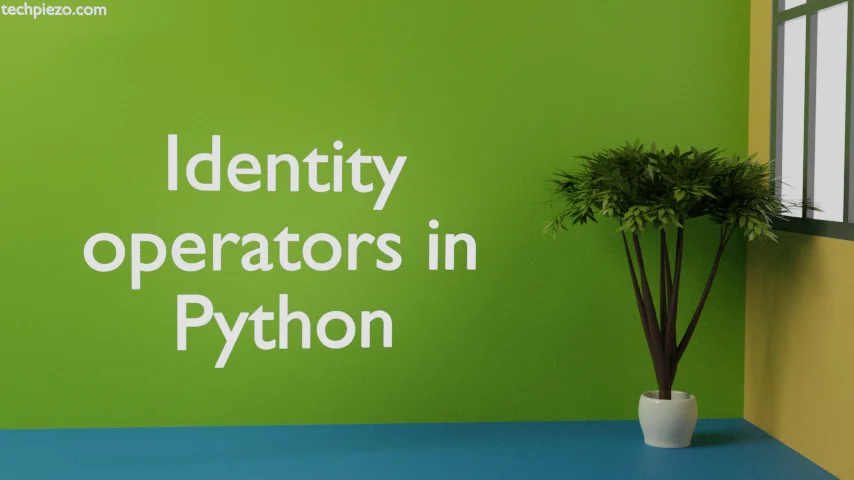
There are mainly two identity operators in Python –
- is operator and,
- is not operator.
These are different from equality (==) and not equal (!=) operators. The identity operators check if the two variables corresponds to the same object and the memory location. We start with is operator first.
is identity operator in Python
As already discussed, the is operator would return with True if the two variables are identical. Which means they have the same object and the memory location. Let’s understand with the help of an example.
We declare a list x, which has the following values assigned –
x = [2,5,6]
And, we assign the values of x in y
y = x
To check if x and y are identical
x is y
It returns with the following,
True
Now, consider a scenario where both the values of list x and y are equal.
x = [2,5,6] y = [2,5,6] x is y
This would return with –
False
Although, both the values are same still it returns with False because x and y variables don’t correspond to the same object and memory location.
is not identity operator in Python
is not is exactly opposite to identity operator – is. Let’s say we two lists x and y –
x = [2,5,6] y = [2,5,6] x is not y
This would return with –
True
Clearly, values are same but not the objects. So, it returns with True. Although x is equal to y value-wise. But, we can’t say they are identical. Now, let’s consider the situation where x and y are identical.
x = [2,5,6] y = x x is not y
It returns with –
False
Reason – because x is y.
In conclusion, we have covered two identity operators in Python here.