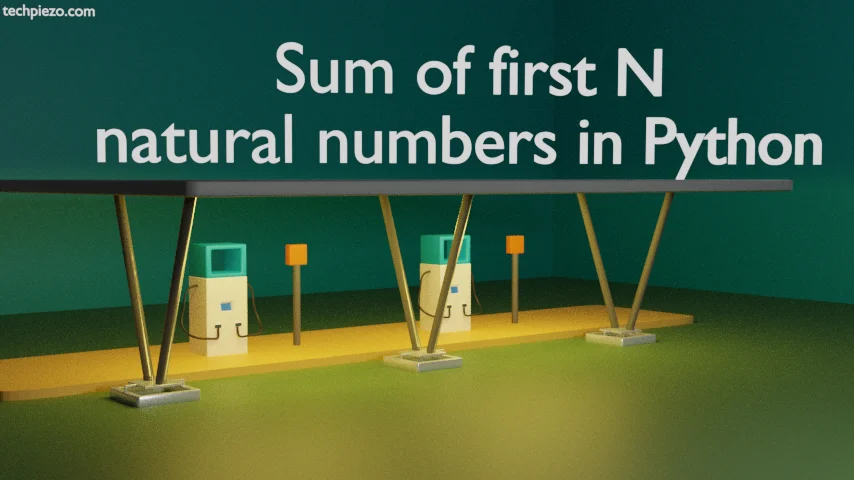
In this article, we cover how to write a Python program that helps us calculate the sum of the first N natural numbers.
Introduction
It is important that we understand what we are about to achieve here. Natural numbers are all positive integers numbers. They do not include decimals, fractions, and negative numbers. So, natural numbers start from 1,2,3 up to……∞.
So, the sum of the first 4 natural numbers will be:
1+2+3+4 = 10
Python program that calculates the Sum of the first N natural numbers
Either we can calculate the sum directly by adding the numbers or we can use the formula. There are numerous other ways as well.
Method I.
num = int(input("Enter the number, N: ")) sum = 0 for i in range(1, num+1): sum += i print(f"The sum of first {num} natural number is: {sum}")
We asked the user to enter the value of N. This value is stored in variable num. The initial value of the variable sum is zero.
Thereafter, we used for loop and range() function. variable i starts with the initial value of 1 and goes up to num+1. And, as the value of i increases, it is added to the variable sum.
Lastly, we used the print() function to view the sum of the first N natural numbers.
Testing the Code:
When we run our code, it prompts us to enter the value of N. And, displays the sum.
Enter the number, N: 5 The sum of first 5 natural number is: 15
Method II.
Alternatively, we can use the formula to calculate the sum of the first N natural numbers.
Sum of first N natural numbers = (N * (N+1)) / 2
num = int(input("Enter the number, N: ")) sum = int((num*(num+1))/2) print(f"The sum of first {num} natural number is: {sum}")
This one is pretty straightforward. Just that, we have used int() here while calculating the sum. We can do away we that if required.
Testing the Code:
It would prompt us to enter the number, N. Let’s say we chose to keep this value 5. It would calculate the return us the required value.
Enter the number, N: 5 The sum of first 5 natural number is: 15
Conclusion
In this article, we have covered how to calculate the sum of the first N natural numbers through a program in Python. Two methods to calculate the sum are covered here. One was through a formula and the other was by calculating the sum directly by using for loop and range() function.
You can read more about Natural numbers: