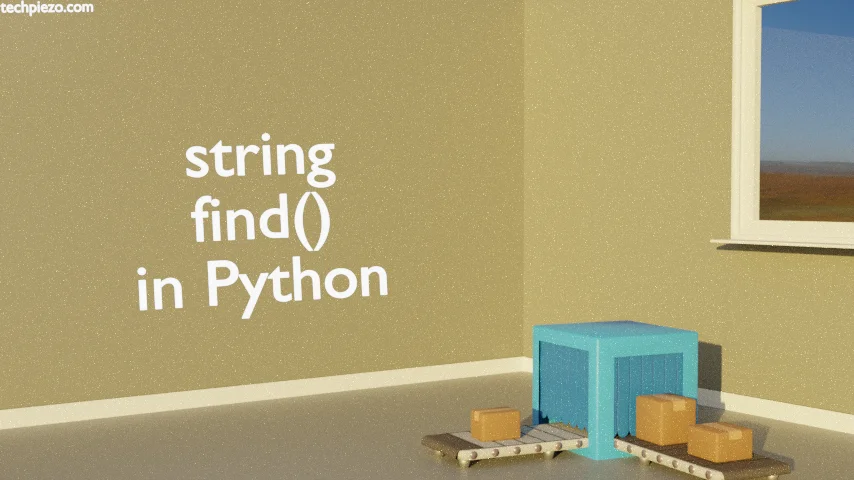
In this article, we would cover string find() method in Python. Sometimes, we need to find a substring which is a part of larger string. When the find() method finds the substring, it returns the lowest index at which substring is found. And, if the substring isn’t there then, it returns with -1.
Following is the syntax of find() –
str.find(sub[, start[, end]])
where,
sub – this will be the substring we are looking for. It is necessary to provide the substring value.
start – an optional argument, position from where the find() should start looking for the substring. If we don’t provide anything, it takes default value as 0.
end – again an optional argument, position at which find() should stop looking for the substring. If we don’t provide anything, it takes default value till end of string index.
string find() in Python
We illustrate it with necessary examples here. In first example, we won’t consider start and end arguments. And, we will consider start and end arguments in second example to see how its going to impact the outcome.
Example I. Let’s say we have a string – “Hello, how are you?” and we are looking for position of “are” substring.
x = "Hello, how are you?" fd = x.find("are") print(fd)
It should return with –
11
What we did here? We stored larger string in variable x. And, we tried to identify the position at which the substring is placed. The result is stored in variable fd, which is printed later.
But, what if substring wasn’t simply there in the larger string –
x = "Hello, how are you?" fd = x.find("bye") print(fd)
This time around, it should return with –
-1
As expected, it didn’t find the relevant substring in the larger string.
Example II. Here, we will take the start and end arguments in consideration while looking for a substring. Continuing with the above example –
Case I.
x = "Hello, how are you?" fd = x.find("are", 2, 9) print(fd)
This would return with –
-1
Since, the find() method couldn’t find any substring between 2 & 9 positions.
Case II. But, this time around we on purpose include the positions where “are” substring could be found –
x = "Hello, how are you?" fd = x.find("are", 7, 12) print(fd)
This would again return with –
-1
Reason: It doesn’t cover the entire string.
Case III. But, if we take values between 7 and 14. It should identify the position of the substring.
x = "Hello, how are you?" fd = x.find("are", 7, 14) print(fd)
This time around, it would return with –
11
In conclusion, we have covered string find() method in Python.