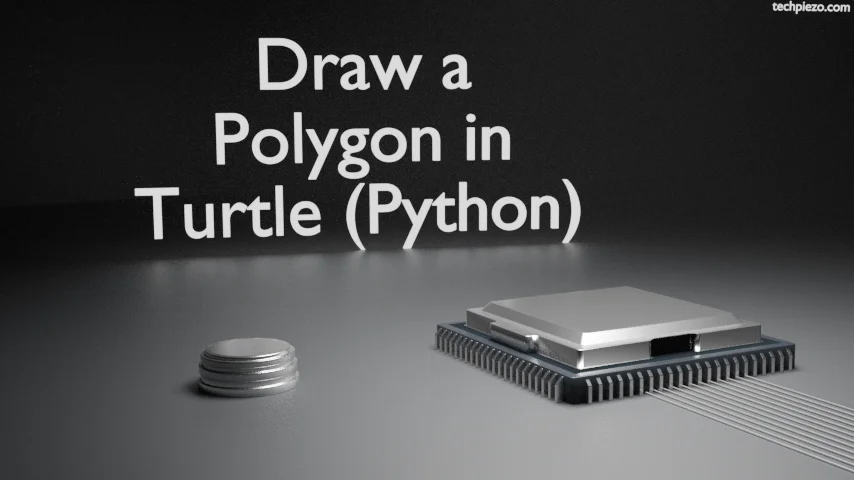
In this article, we would discuss how to draw a polygon in Turtle. Turtle is basically an inbuilt module in Python. With the help of Turtle we can draw various shapes.
It mainly utilizes tkinter for the graphics. Thus, we need to have tkinter along-with Python.
So, if you don’t have tkinter then, it would throw an error –
ModuleNotFoundError: No module named 'tkinter'
If you already have IDLE (Integrated Development and Learning Environment). Then, tkinter is already installed. But, if its not then follow the instructions below.
Note: Following operations would require you to have superuser privileges. In case you don’t have one, then we advise you to contact your System Administrator.
sudo apt update
Since, the package is available through standard Ubuntu repository. Therefore, updating the repository helps. Next, to install tkinter –
sudo apt install python3-tk
Draw a polygon in Turtle (Python)
With all set, we move towards the concept we are about to utilize to draw a polygon of any shape with Turtle. Firstly, we need to know the exterior angle of a polygon. This is pretty easy to find if we know the number of sides of our polygon.
So, exterior angle of a polygon = 360/number of sides
Understand it with the help of an example –
If the number of sides is 3 (i.e. a triangle), then the exterior angle of a triangle = 360/3. So, the exterior angle of a triangle is 120 degrees.
Furthermore, all we need to do here is get the number of sides from a user. Divide the number of sides with 360 degrees to know the exterior angle of a polygon. And, then use for loop and forward() and right() methods from Turtle module to draw the required shape.
So, this is how the code should be –
# Draw a polygon in turtle import turtle # Number of sides of the polygon s = int(input("Enter the number of sides of the polygon : ")) # Length of sides of the polygon l = int(input("Enter the length of sides of the polygon : ")) for i in range(s): turtle.forward(l) turtle.right(360/s)
here, turtle.forward(l) – it moves and draws l pixels in the direction it is facing,
turtle.right(360/s) – it rotates in place 360/s. For instance, for a triangle it would rotate 120 degrees.
We use import turtle to import the builtin module. for loop to make a particular instruction set to execute ‘s’ number of times.
Rest of the code is self-explanatory.
In conclusion, we have discussed how to draw a polygon in Turtle.