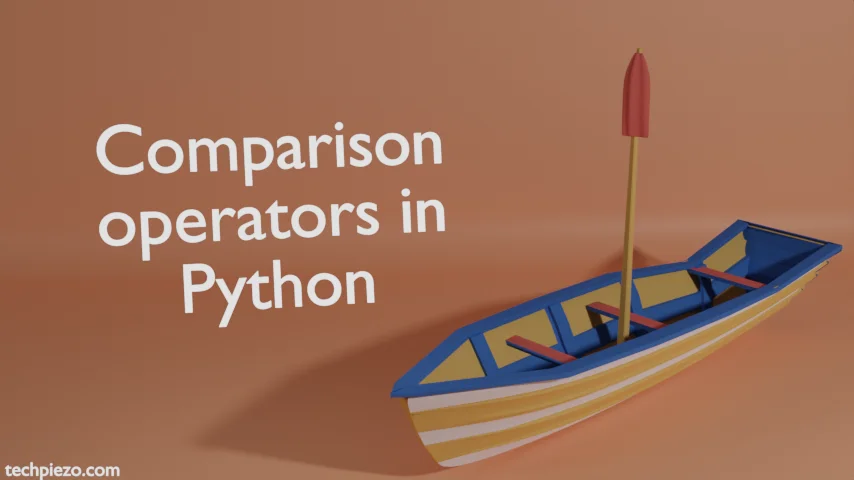
A Python code often involves comparing values stored in variables. This is made possible through comparison operators. For instance, with if..else statements. If some condition satisfies, it executes A otherwise B. There are six comparison operators in Python –
- Equal,
- Not equal,
- Less than,
- Greater than,
- Greater than or equal to and,
- Less than or equal to.
We will discuss each of these with relevant examples next.
Comparison operators in Python
A. Equal operator (==) – We first start with Equal comparison operator. In simple terms, if value on the Left hand side is equal to Right hand side then, it would return with True. Otherwise it would be False. For instance,
10 == 10 "z" == "z"
It would return with True. But, it returns with False for the following condition –
"z" == "26"
B. Not equal operator (!=) – It is used to check if two values are equal or not. For instance –
10 != 11 "z" != "x"
For the above it would return with True – as 10 is not equal to 11 and z is not equal to x. But, a False would result in if we use the following –
10 != 10
C. Less than (<) – If value on the left hand side is less than right hand side one. Then, it would result in True. To compare string values, it checks for first letter and if the first letter is same. Then, it compares subsequent letters in the word. For instance,
"a" < "c" 10 < 20 "Hello" < "Text"
All the above conditions would result in True.
D. Greater than (>) – If the value on left hand side is greater than the right hand side then, it results in True. Otherwise, it returns with False. For instance –
20 > 10 "c" > "a" "Text" > "Hello"
Above code would return with True. String value comparison we have already covered in Section C.
E. Greater than or equal to (>=) – If the left hand side is greater than or equal to right hand side value. It would result in True. Notice that, with Greater than (>) – it was just one condition we had to check. But, with Greater than or equal to – we need to check for two conditions. Following code would result in True.
20 >= 10 20 >= 20
F. Less than or equal to (<=) – If the left hand side is less than or equal to right hand side value. It would result in True. Again, we check two conditions – less than (<) and equal to (=).
10 <= 10 10 <= 20
The above code results in True.
In conclusion, we have discussed Comparison operators in Python here.