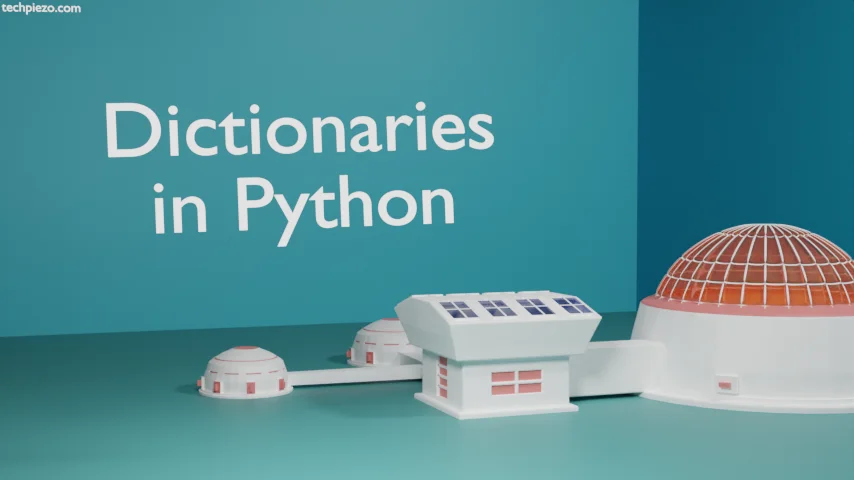
In this article, we would cover Dictionaries in Python. We use Dictionaries to store data as a set of key:value pairs. Every key is has a corresponding value. Furthermore, in the dictionary – keys have to be unique while we can have duplicate values. In other words, two or more values in the dictionary can exist. But, the same can’t be said for the keys, they always have to be unique.
We use curly brackets {} to declare dictionaries. Each key:value pair is separated by a comma (,). In addition to, if we use the same key again in the dictionary then the old value associated with that particular key is gone. We can only have the latest value associated with that particular key.
So, with basics done – we now see how to declare a dictionary –
mydict = {"status": "draft", "post": 354, "name": "TechPiezo"}
Here, mydict is the name of our dictionary. status, post and name are the keys. And, draft, 354 and TechPiezo are the corresponding values.
To help you understand the concept better, we discuss it with appropriate examples next.
Dictionaries in Python
Example I. Print dictionary – Let’s say we have dictionary – mydict
mydict = {"status": "draft", "post": 354, "name": "TechPiezo"}
To print the dictionary, we can use print() method –
print(mydict)
It would return with –
{'status': 'draft', 'post': 354, 'name': 'TechPiezo'}
Now, what if we want to print a value using an associated key –
print(mydict['post'])
This would return with –
354
Here, we used the key ‘post’ to get the corresponding value – 354.
Example II. Check the length of the dictionary. It would basically return with the number of key:value pairs in the dictionary. We continue with the above example –
mydict = {"status": "draft", "post": 354, "name": "TechPiezo"}
To check for the length of dictionary –
len(mydict)
It would return with –
3
Example III. Add an item i.e. key:value pair in the dictionary. If we want to add the following key:value pair to mydict –
published: yes
So, to add the above key:value pair we can use the following code –
mydict["published"] = "yes"
To see the changes –
print(mydict)
It would return with –
{'status': 'draft', 'post': 354, 'name': 'TechPiezo', 'published': 'yes'}
The above outcome is self-explanatory.
In conclusion, we have discussed Dictionaries in Python.