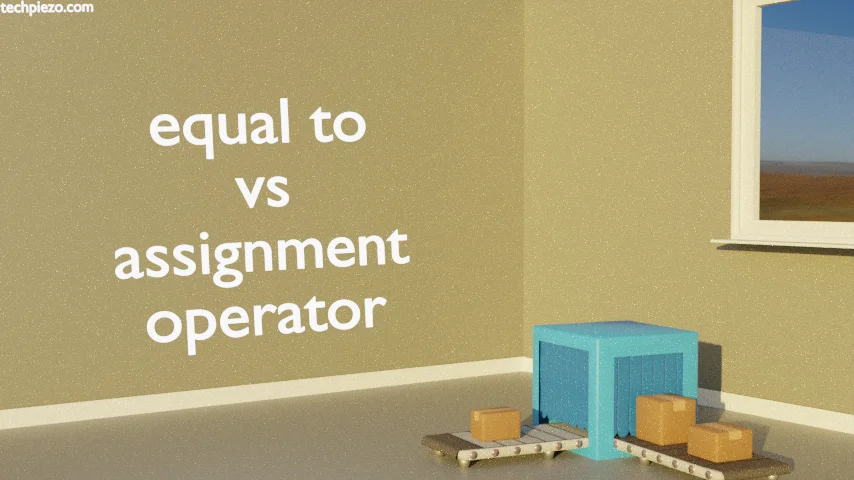
In this article, we would discuss the difference between equal to (==) and assignment operator (=) in Python. In programming languages, we need to assign values to the variables this is achieved through assignment operator. Furthermore, to compare values stored in variables we use comparison operators. Want to know about comparison operators in Python? equal to comes under the category of comparison operators.
If you have just started with Python then it may look confusing in the beginning. But, these are pretty easy to use once we understand what we are trying to achieve with both these operators. So, we start with the basic introduction followed by relevant examples.
Difference between equal to (==) and assignment operator (=) in Python
Firstly, equal to (==) operator – as already discussed it is a comparison operator. It is used to check if two values/variables are equal. For instance, string “abc” is equal to string “abc” but not equal to string “xyz”. If the condition satisfies then, it returns with True otherwise False. In addition to, it won’t always be plain True/False outcome. At times, we would ask the interpreter to execute a specific code if a condition satisfies otherwise something else. Even for those cases, the equal to (==) operator works just fine.
Now, understand it with couple of examples next. We compare an integer with integer and then string with a string.
1 == 1 "abc" == "abc"
For both, it would return with True.
1 == 2 "abc" == "xyz"
As expected, it returns with False. We go a step further and use if..else statement.
x = 1 if (x==1): print("x equals 1") else: print("x is not equal to 1")
It would return with –
x equals 1
Note: Please take care of indentation in above code otherwise it would result in an error.
Here, if..else statement checks whether x is equal to 1 or not. And, shows the outcome accordingly. If you want to know more about if..else statement in Python .
Secondly, assignment (=) operator – these are used to assign values to the variable. If we want to write an efficient and clutter-free code then, we have to assign values to the variables. And, these variables can later be used to perform various operations. So, this is how it works –
x = "abc" y = 1 print("Value of x: ", x) print("Value of y: ", y)
It would return with –
Value of x: abc Value of y: 1
Clearly, we assigned some value to x and y variables. Later, these we printed using print() method.
In conclusion, we have covered difference between equal to and assignment operators operator in Python here.