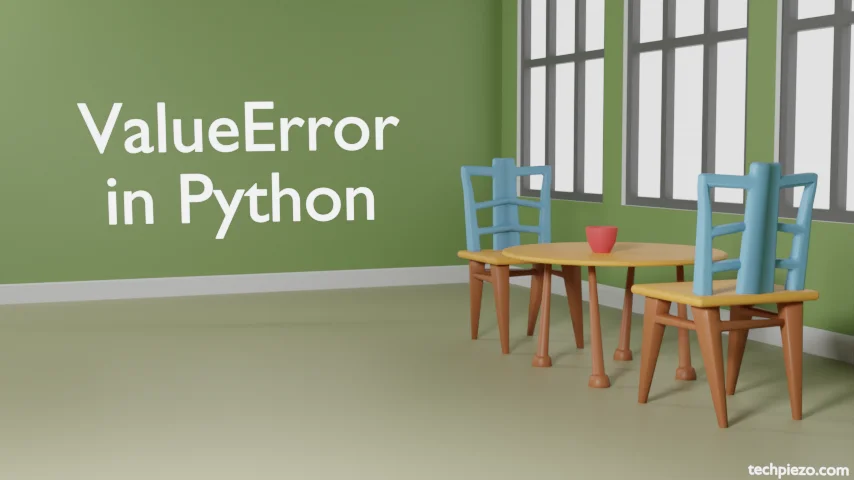
In this article, we would cover ValueError in Python. Sometimes, we just pass an argument of right type to a function. But, completely miss to pass the appropriate value. In such cases, we get ValueError in Python.
We understand the cases wherein we would get a ValueError through couple of examples.
ValueError in Python
Example I. Certain mathematical operations require only positive integer values. One of such mathematical operation could be finding the factorial of number. In Python, we need to first import the math module.
import math print(math.factorial(-10))
Interpreter would return with –
ValueError: factorial() not defined for negative values
But, same can’t be said for value (-10.2). Because, then it would throw TypeError. Try it as well –
import math print(math.factorial(-10.2))
It returns with Error type along with the reason –
TypeError: 'float' object cannot be interpreted as an integer
Example II. ValueError also occurs when we provide too many values to unpack –
x = y = z = 1 x, y = y, x, z
It would return with –
ValueError: too many values to unpack (expected 2)
It expected two values, but we have provided three.
There are numerous other conditions which would result in a ValueError. But, we hope you have got the basic notion.
Handle ValueError exceptions
So, we now focus on handling exceptions. We continue with the Example I here. As already discussed, factorial() method won’t accept negative integer values. Therefore, we can easily handle any arising ValueError exception.
We utilize try except block to handle exceptions. So, the code in Example I can be rewritten as –
import math try: print(math.factorial(-10)) except ValueError: print("Use only positive integer values")
Instead of ValueError, it would return with –
Use only positive integer values
In conclusion, we discussed ValueError with two examples here. Then, how we can handle it through try except block was also covered.