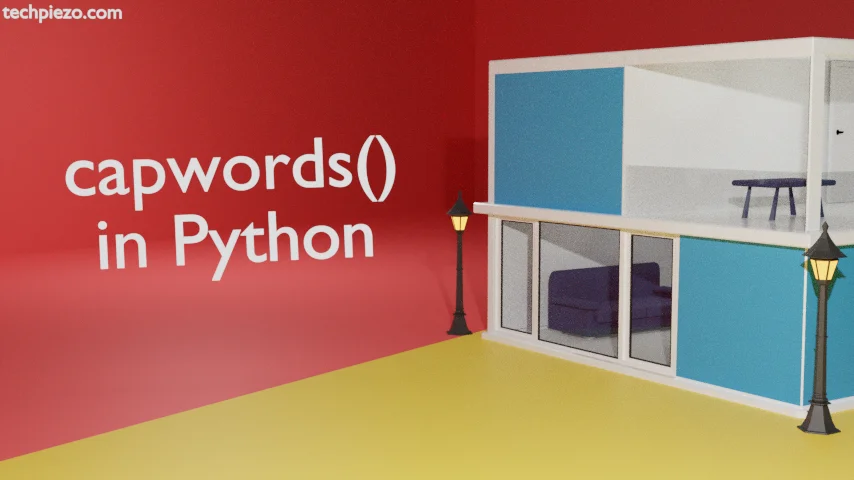
In this article, we would cover capwords() function in Python. The capwords() basically converts the first character of every word in a string to uppercase. The outcome is pretty similar to the title() method we have already discussed it. It basically does three operations –
- It splits the entire string to words using str.split(),
- Then, it capitalizes the first character of every word through str.capitalize(),
- Lastly, it joins those words through str.join().
Following is the syntax for capwords() –
string.capwords(s, sep=None)
Now, understand the concept more through couple of examples.
capwords() in Python
Example I. First we need to import string module.
import string
Next, let’s say we have a string x,
x = "hello, how are you?"
To convert first character of every word to uppercase, we use capwords() function and store the outcome in variable y,
y = string.capwords(x)
Furthermore, to display the result through print() method –
print(y)
It would return with –
Hello, How Are You?
We haven’t used a separator here. Apart from that, it converts the first character of every word to uppercase.
Example II. We use a separator in this example. Again, import a string module.
import string x = "hello, how are you?" y = string.capwords(x, sep='o') print(y)
Observe here that, we have used a separator ‘o‘. This time around, it would return with –
Hello, hoW are yoU?
Every time it encounters ‘o‘, it just converts the following character to uppercase.
Example III. Here, we will see how it affects when it encounters integers values as first character of a word.
import string x = "4ello, how 4re you?" y = string.capwords(x) print(y)
We chose to skip the separator. It would return with –
4ello, How 4re You?
The outcome is self-explanatory.
In conclusion, we have discussed capwords() function in Python.